One of the many popular feature of IBM MobileFirst SDK is the ability to capture client-side logs from mobile devices out in the wild in a central location (on the server). That means you can capture information from devices *after* you have deployed your app into production. If you are trying to track down or recreate bugs, this can be incredibly helpful. Let’s say that users on iOS 7.0, specifically on iPhone 4 models are having an issue. You can capture device logs at this level of granularity (or at a much broader scope, if you choose).
The logging classes in the MobileFirst Platform Foundation are similar in concept to Log4J. You have logging classes that you can use to write out trace, debug, info, log, warn, fatal, or error messages. You can also optionally specify a package name, which is used to identify which code module the debug statements are coming from. With the package name, you’ll be able to see if the log message is coming from a user authentication manager, a data receiver, a user interface view, or any other class based upon how you setup your loggers. Once the log file reaches the specified buffer size, it will automatically be sent to the server.
On the server you can setup log profiles that determine the level of granularity of messages that are captured on the server. Let’s say you have 100,000 devices consuming your app. You can configure the profiles to collect error or fatal messages for every app instance. However, you probably don’t want to capture complete device logs for every app instance; You can setup the log profiles to only capture complete logs for a specific set of devices.
As an example, take a look at the screenshot below to see how you can setup log collection profiles:
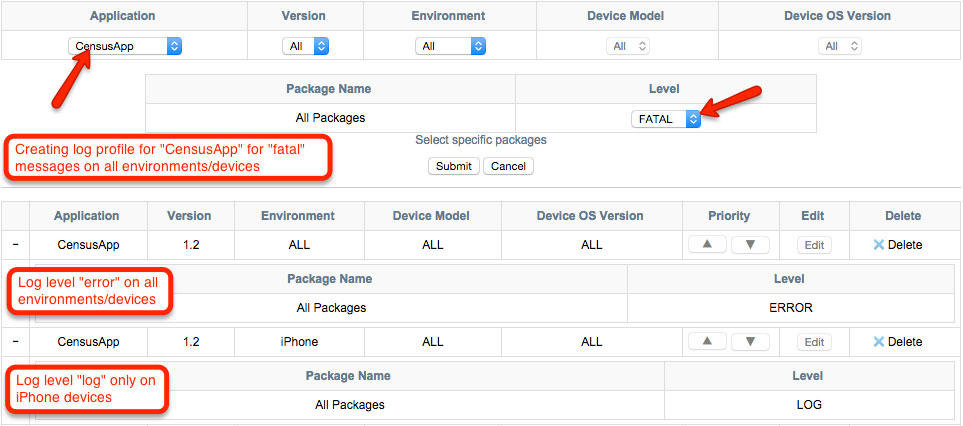
When writing your code, you just need to create a logger instance, then write to the log.
If you’re curious when you might want a trace statement, vs. a log statement, vs. a debug statement, etc… Here is the usage level guidance from the docs:
- Use TRACE for method entry and exit points.
- Use DEBUG for method result output.
- Use LOG for class instantiation.
- Use INFO for initialization reporting.
- Use WARN to log deprecated usage warnings.
- Use ERROR for unexpected exceptions or unexpected network protocol errors.
- Use FATAL for unrecoverable crashes or hangs.
For hybrid apps, you use the WL.Logger class in JavaScript:
[js]var logger = WL.Logger.create({pkg: ‘mynamespace.mymodule’});
logger.trace(‘trace’, ‘another mesage’);
logger.debug(‘debug’, [1,2,3], {hello: ‘world’});
logger.log(‘log’, ‘another message’);
logger.info(‘info’, 1, 2, 3);
logger.warn(‘warn’, undefined);
logger.error(‘error’, new Error(‘oh no’));
logger.fatal(‘fatal’, ‘another message’);[/js]
For native iOS apps, you will use the OCLogger class:
[objc]OCLogger *logger = [OCLogger getInstanceWithPackage:@"UserManager"];
[logger trace:@"this is a trace message"];
[logger debug:@"this is a debug message"];
[logger log:@"this is a log message"];
[logger info:@"this is an info message"];
[logger warn:@"this is a warning message"];
[logger error:@"this is an error message"];
[logger fatal:@"this is a fatal message"];[/objc]
For native Android apps, you will use the com.worklight.common.Logger class:
[java]private final static Logger logger = Logger.getLogger(MyClass.class.getName());
logger.trace(‘trace mesage’);
logger.debug(‘debug message’);
logger.log(‘log message’);
logger.info(‘info message’);
logger.warn(‘warn message’);
logger.error(‘error – OH NOES!’);
logger.fatal(‘fatal – Oops, you broke it’);[/java]
Then on the server, you can go into the analytics dashboard and access complete logs for a device, or search through all client-side logs with the ability to filter on application name, app versions, log levels, package name, environment, device models, and OS versions within an optional date range, and with the ability to search for keywords in the log message.
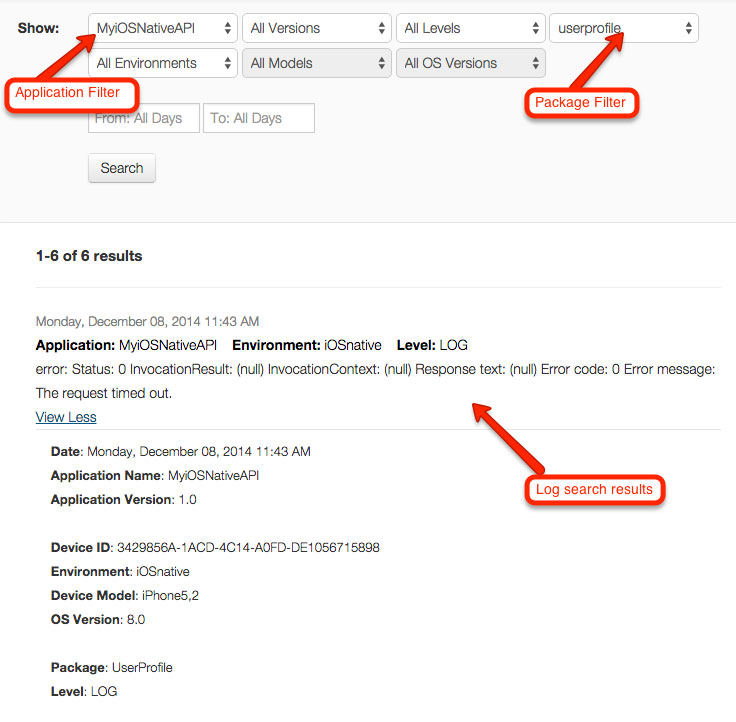
For a complete reference and additional detail, be sure to check out the latest docs on client side logging with the MobileFirst platform.