IBM MobileFirst Foundation (also known as IBM Worklight) is a middleware solution for developing mobile applications. Out of the box, Worklight enables security and authentication, device management, encrypted storage, operational analytics, simple cross platform push notifications, remote logging, data access, and more…
Historically, most people think that Worklight is just for creating hybrid mobile (HTML-powered) applications. While this is one of the powerful workflows that Worklight enables, it’s also the proverbial “tip of the iceberg”. Worklight does a lot more than just provide the foundation for secure hybrid applications. Worklight also provides a secure foundation for native applications, and can be easily incorporated to any existing (or new) native app.
In this post, I’ve put together a video series to take a look at just how easy it is to setup Worklight in an existing iOS native application to provide remote log collection, application management, and more. Read on for complete detail, or check out the complete multi-video playlist here.
The existing app that I’ll be integrating is based on an open source Hacker News client, which I downloaded from GitHub. Check out the video below for a quick introduction to what we’ll be building.
If you want, you can leverage the Worklight IDE – a set of developer tools built on top of the Eclipse development environment. If you don’t want to use Eclipse, that’s OK too – Worklight can be installed and configured using the Worklight command line interface (CLI) and you can leverage any developer tools that you’d like to build your applications. Want to tie into Xcode ? No problem. I’ll be using the Worklight CLI to setup the development environment in this series.
Setting up the Worklight Server
The Worklight server is the backbone for providing Worklight features. App managment, remote logging, operational & network analtics, and all of the Worklight features require the server, so the first thing that you need to do is setup the server for our environment. Check out the next video, which will guide you through setting up a Worklight server/project using the CLI.
First things first, you have to start the Worklight server. If you haven’t already configured a Worklight server, run the create-server command to perform the initial setup – this only has to be run once ever.
[shell]wl create-server[/shell]
Now that the server is setup, we need to create a Worklight project. For this walkthrough, I’ll just call it “MyWorklightServer”:
[shell]wl create MyWorklightServer[/shell]
Next, go into the newly created project directory and go ahead and start it.
[shell]cd MyWorklightServer
wl start[/shell]
Once the server is started, add the iOS platform:
[shell]wl add api[/shell]
You will be prompted for an API name. This can be anything, but you should probably give it a meaningful name that identifies what the API will be used for. In this walkthrough I specify the name “MyiOSNativeAPI”.
Next you will be prompted to select a platform, select “iOS”
Next, build the project, and then deploy it to the server:
[shell]wl build
wl deploy[/shell]
Next, launch the Worklight console to view that the project has been deployed and the native API has been created. The console will launch in the system web browser.
[shell]wl console[/shell]
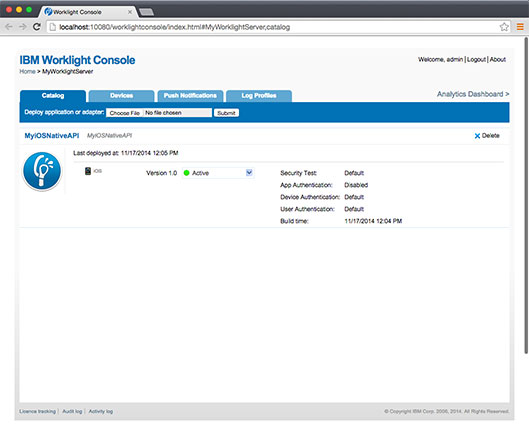
Be sure to check out the Worklight CLI documentation for complete detail on the CLI commands.
Xcode Integration
Next we need to setup the Xcode project to connect to the newly created Worklight server. If you’re adding Worklight to a new Xcode project, or an existing Xcode project, the preparation steps are the same:
- Add Worklight files to your Xcode project
- Add framework dependencies
- Add the -ObjC linker flag
This next video walks through configuration of your Xcode project and connecting to the Worklight server (which we will cover next):
In the Xcode project navigator, create a folder/group for the Worklight files, then right click or CTRL-click and select “Add Files to {your project}”…
Next, navigate to the newly created MyiOSNativeAPI folder and select the worklight.plist file and WorklightAPI folder, and click the “Add” button.
Once we’ve added the files to our Xcode project, we need to link the required framework/library dependencies:
- CoreData.framework
- CoreLocation.framework
- MobileCoreServices.framework
- Security.framework
- SystemConfiguration.framework
- libstdc++.6.dylib
- libz.dylib
Next, In the Xcode project’s Build Settings search for “Other Linker Flags” and add the following linker flag: “-ObjC”.
If you’d like additional detail, don’t miss this tutorial/starter app by Carlos Santana: https://github.com/csantanapr/wl-starter-ios-app
Connecting to the Worklight server
Connecting to the Worklight server is just a few simple lines of code. You only have to implement the WLDelegate protocol, and call wlConnectWithDelegate, and the Worklight API handles the rest of the connection process. Check out the video below to walk through this process:
Implement the WLDelegate protocol:
[objc]//in the header implement the WLDelegate protocol
@interface MAMAppDelegate : UIResponder <UIApplicationDelegate,
WLDelegate> {
//in the implementation, add the protocol methods
-(void)onSuccess:(WLResponse *)response {
}
-(void)onFailure:(WLFailResponse *)response {
}[/objc]
Connect to the Worklight Server:
[objc][[WLClient sharedInstance] wlConnectWithDelegate: self];[/objc]
Next, go ahead and launch the app in the iOS Simulator.
You’re now connected to the Worklight server! At this point, you could leverage app management and analytics through the Worklight Console, or start introducing the OCLogger class to capture client side logging on the server.


Remote Collection of Client Logs
Once you’re connected to Worklight, you can start taking advantage of any features of the client or server side APIs. In this next video, we’ll walk through the process of adding remote collection of client app logs, which could be used for app instrumentation, or for debugging issues on remote devices.
On the server, you’ll need to add log profiles to enable the capture of information from the client machines.

On the client-side, we just need to use the OCLogger class to make logging statements. These statements will be output in the Xcode console for local debugging purposes. If a log profile has been configured on the server, these statements will also be sent to the Worklight server.
[objc]OCLogger *logger = [OCLogger getInstanceWithPackage:@"MyAppPackageName"];
[OCLogger setCapture:YES];
[OCLogger setAutoSendLogs:YES];
//now log something
[logger log:@"worklight connection success"];
[logger error:@"worklight connection failed %@", response.description];
[/objc]
For complete reference on client side logging, be sure to review the Client-side log capture API documentation.
App Management & Administration
Out of the box, Worklight also provides for hassle-free (and code-free) app management. This enables you to set notifications for Worklight client apps and disable apps (cutting off access to services and providing update URLs, etc..). This next video walks you through the basics of app management.
Be sure to check out the complete documentation for app management for complete details.
All done, right?
At this point, we’ve now successfully implemented Worklight server integration into a native iOS app. We have remote collection of client-side logs, we can leverage app management, and we can collect operational analytics (including platforms, active devices, and much more).
If you don’t want to leverage any more Worklight features, then by all means, ship it! However, Worklight still has a LOT more to offer.
Exposing Data Through Adapters
Worklight also has the ability to create adapters to expose your data to mobile clients. Adapters are written in JavaScript and run on the server. They help you speed up development, enhance security, transform data serialization formats, and more.
In the next two videos we will walk through the process of collecting information inside the native iOS application and pushing that into a Cloudant NoSQL data base (host via IBM Bluemix services).
Cloudant databases have a REST API of their own, so why use a Worklight Adapter? For starters, Worklight becomes your mobile gateway. By funneling requests through Worklight, you are able to capture analytic data for every server invocation, and Worklight gives us the ability to control access to the enterprise. Worklight gives you the capability to cut off mobile access to the backend at any time, just by changing the API status.
Now let’s take a look at the process for exposing the Cloudant database through a Worklight Adapter:
Once the data adapter has been configured, it is simple to invoke the adapter procedures to get data into or out of the your applications.
This next video covers the process of pushing data into the Cloudant database adapter from the native mobile client application:
Once again, you will have to implement the WLDelegate protocol to handle success/error conditions, and the procedure invocation is implemented using the WLClient invokeProcedure method:
[objc]NSMutableDictionary *userProfile = [[NSMutableDictionary alloc] init];
[userProfile setValue:self.email.text forKey:@"email"];
[userProfile setValue:self.firstName.text forKey:@"firstName"];
[userProfile setValue:self.lastName.text forKey:@"lastName"];
WLProcedureInvocationData * invocationData = [[WLProcedureInvocationData alloc] initWithAdapterName:@"CloudantAdapter" procedureName:@"putData"];
invocationData.parameters = @[userProfile];
[[OCLogger getInstanceWithPackage:@"UserProfile"] log:@"sending data to server"];
[[WLClient sharedInstance] invokeProcedure:invocationData withDelegate:self];[/objc]
It is as simple as that.
IBM MobileFirst Foundation (aka Worklight) is for more than just hybrid app development. It is a secure platform to streamline your enterprise application development processes, including everything from encryption, security and authentication, to operational analytics and logging, to push notifications, and much more, regardless of whether you’re targeting hybrid app development paradigms, or native iOS, native Android, or native Windows Phone projects.